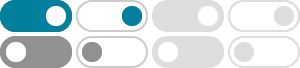
How to split by comma and strip white spaces in Python?
I have some python code that splits on comma, but doesn't strip the whitespace: >>> string = "blah, lots , of , spaces, here " >>> mylist = string.split(',') >>> print mylist ['blah', ' lots ', ' of ', ' …
Python - Removing \n when using default split ()? - Stack Overflow
Sep 22, 2016 · The default str.split targets a number of "whitespace characters", including also tabs and others. If you do str.split(' '), you tell it to split only on ' ' (a space). You can get the …
Python split() without removing the delimiter - Stack Overflow
re.split (r" (?<=> (?!$))", '<html><head>') directly gives the answer. This way it can be handled by playing with regex look-arounds. s = [e+d for e in line.split(d) if e] That works perfectly... but I …
Python String split() Method - W3Schools
The split() method splits a string into a list. You can specify the separator, default separator is any whitespace. Note: When maxsplit is specified, the list will contain the specified number of …
Split a String and remove the Whitespace in Python | bobbyhadz
Apr 8, 2024 · Use the str.split() method to split the string into a list. Use a list comprehension to iterate over the list. On each iteration, use the str.strip() method to remove the leading and …
10+ Ways To Remove Unwanted Spaces From Your Python Strings
Feb 17, 2025 · Python offers a range of built-in functions and methods to tackle this problem. Here are some of the most effective ways to remove unwanted spaces from your strings. 1. …
Python String split() - GeeksforGeeks
Jun 20, 2024 · split () function operates on Python strings, by splitting a string into a list of strings. It is a built-in function in Python programming language. It breaks the string by a given …
How to Split a String in Python
You split a string by spaces in Python using .split() without arguments. Python’s .split() method can split on custom delimiters when you pass a character or string as an argument. You limit …
Split a string in Python (delimiter, line break, regex, and more)
Aug 9, 2023 · This article explains how to split a string by delimiters, line breaks, regular expressions, and the number of characters in Python. Refer to the following articles for more …
Top 5 Methods to Split by Comma and Remove Whitespaces in Python
Dec 5, 2024 · Explore effective techniques to split strings by commas in Python while removing unwanted whitespace. Learn five distinct methods to streamline your string manipulation.